Add a self-hosted player (Web)
Learn how to embed a self-hosted player on your site
The self-hosted player gives you full control over player versioning and hosting. This approach is ideal if you need to pin to a specific player version, support offline environments, or manage updates manually.
This article explains how to implement a self-hosted player on your site.
Prerequisites
Item | Description |
---|---|
Media | Content to play within the player
For best results, use media or playlists managed in the dashboard. This approach ensures access to optimized renditions, thumbnails, and bandwidth handling. Follow these steps to obtain the platform-hosted media or playlist URL: Single Media
Playlist
You can also host your media on your own servers. However, self-hosting requires manual optimization for renditions, thumbnails, and adaptive streaming. |
Enterprise License | License to access advanced features
An Enterprise license is required for advanced features. Please contact our team for information. |
Add a self-hosted player
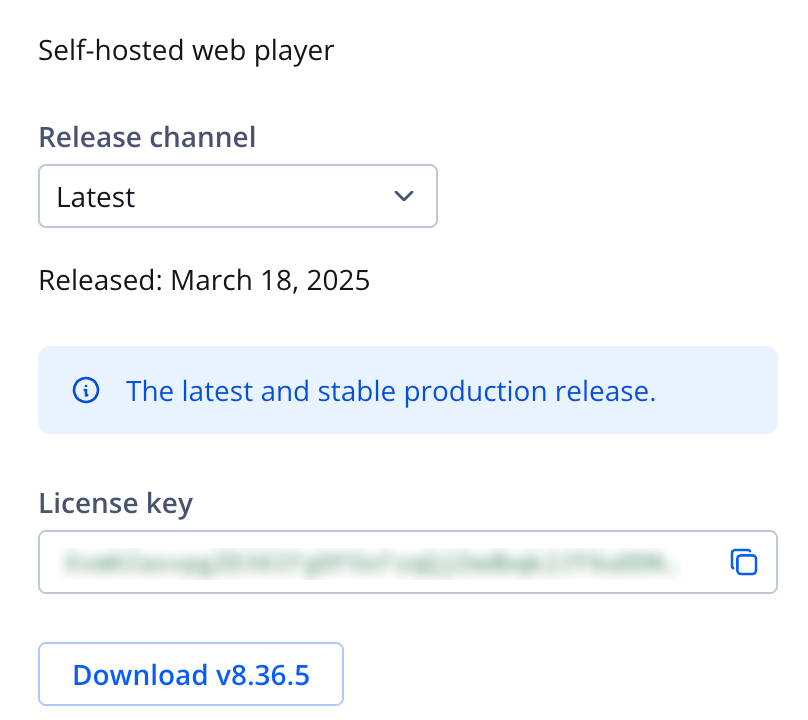
Self-hosted web player section
Follow these steps to access the player code, which you can host anywhere within your ecosystem, and add the player to your website:
- On the Properties page, click the name of a property. The property settings appear.
- Under Network & setup files, click Player setup files. The Player setup files page appears.
- Under Self-hosted web player, select a Release channel.
- Copy and save the License key for the player library.
- Click the Download v8.xx.x button to download the player library to your device.
- Unzip the downloaded file.
- Rename and upload the unzipped library folder to your server.
When renaming the folder, remove the periods from the folder name.
-
Within the
<head>
of your site page, copy and paste the URL of the player library and your license key within a<script>
element.<script src="https://www.yourdomain.com/{PATH_TO_PLAYER_FOLDER}/jwplayer.js"></script> <script>jwplayer.key="{LICENSE_KEY}"</script>
-
Within the
<body>
of your page, define a named<div>
for the player and add the playersetup()
. The named<div>
determines where the player will appear on the page. Thesetup()
embeds an instance of the player with specified options.<div id="myElement"></div> <script> jwplayer("myElement").setup(); </script>
-
Add the player behaviors and customizations:
- On the Players page, click the name of the player you created and customized.
- On the View JSON tab, click
(copy icon) to copy the configuration JSON.
- Paste the JSON into
setup()
.
<div id="myElement"></div> <script> jwplayer("myElement").setup( <!-- Behaviors and customizations JSON from the dashboard β-> {player_json} ); </script>
You can learn more about each of these options and configure them in detail.
-
Add media to
setup()
:- playlist (JSON/RSS): Configures the player to load a JSON or RSS playlist URL
- playlist (array): Configures the player to load a self-hosted media file or a specific JWP Platform media rendition
<div id="myElement"></div> <script> jwplayer("myElement").setup( {<!-- Behaviors and customizations JSON from the dashboard β-> <!-- Media β-> "playlist": "{json_or_rss_url}" } ); </script>
<!-- Example: Load a self-hosted media file or specific platform-hosted media rendition --> <div id="myElement"></div> <script> jwplayer("myElement").setup( {<!-- Behaviors and customizations JSON from the dashboard β-> <!-- Media β-> "playlist": [{ "file": "{media_resource_url}" }] } ); </script>
When your viewers visit the page, they can click the play icon and watch your content.
if an issue occurs, review the full code example and troubleshoot your player setup.
Most cloud-hosted setups donβt require additional coding. If you have a unique use case, refer to the Advanced Topics articles in the side navigation for additional customization guidance.
Full Code Example
<!DOCTYPE html>
<html lang="en">
<head>
<script src="https://www.yourdomain.com/{PLAYER_FOLDER}/jwplayer.js"></script>
<script>
jwplayer.key = "{LICENSE_KEY}";
</script>
</head>
<body>
<div id="myElement"></div>
<script>
jwplayer("myElement").setup({
advertising: {
admessage: "This video will resume in xx seconds",
adscheduleid: "nmQspR5F",
autoplayadsmuted: false,
cuetext: "Advertisement",
enablePPS: false,
endstate: "suspended",
outstream: false,
preloadAds: false,
skipmessage: "Skip ad in xx seconds",
vpaidcontrols: false
},
aspectratio: "16:9",
autoPause: {
viewability: false
},
autostart: false,
captions: {
backgroundColor: "#000000",
backgroundOpacity: 75,
color: "#ffffff",
edgeStyle: "dropshadow",
fontFamily: "sans-serif",
fontOpacity: 100,
fontSize: 15,
windowColor: "#000000",
windowOpacity: 0
},
controls: true,
displayHeading: false,
displaydescription: true,
displaytitle: true,
floating: {
mode: "never"
},
generateSEOMetadata: false,
height: 270,
include_compatibility_script: false,
interactive: false,
intl: {
en: {
advertising: {
admessage: "",
cuetext: "",
skipmessage: ""
},
sharing: {
heading: "Share Video"
}
}
},
logo: {
hide: false,
position: "top-right"
},
mute: false,
pipIcon: "disabled",
playbackRateControls: false,
preload: "none",
related: {
onclick: "play"
},
repeat: false,
sharing: {
code: "",
sites: []
},
skin: {
controlbar: {
background: "rgba(0, 0, 0, 0)",
icons: "rgba(255, 255, 255, 0.8)",
iconsActive: "#ffffff",
text: "#ffffff"
},
menus: {
background: "#333333",
text: "rgba(255,255,255,0.8)",
textActive: "#FFFFFF"
},
timeslider: {
progress: "#f2f2f2",
rail: "rgba(255, 255, 255, 0.3)"
},
tooltips: {
background: "#FFFFFF",
text: "#000000"
}
},
stretching: "uniform",
width: "100%",
playlist: "{json_or_rss_url}"
});
</script>
</body>
</html>
Updated 2 months ago