Android Styling Guide (Android)
The JWP Android SDK enables you to customize many components of the JWP player UI. The sections below describe, in detail, common use cases for styling the JWP player and how to accomplish each styling update, step-by-step.
While there are many options described below that allow for simple customization of the existing player UI, broad or fundamental changes would most often best be handled by a custom UI. You can accomplish this by building your own
View
and adding it to theJWPlayerView
.
Requirements
You must use JWP SDK 4.5.0 and above.
Best Practice App
You can find a best practice app that styles the player UI here for inspiration and a working example.
Viewing the default JWP resource definitions
In certain scenarios, it is helpful to have access to the original design resources for reference, such as reviewing the default style definitions so you know what properties you must also use in your style definition. You may also want to locate whatโs defined in the drawables used so you can either customize the color or replace the drawable with something similar.
Accessing resources when using the AAR
- Open Android Studio.
- Go to the location in the project where the .aar files exist (for example, ex. app/libs)
- Double click the jwplayer-core module, you should now see the contents of the module.
- Expand the res/values folder and click values.xml.
- You now have access to the original style definitions used in the SDK.
Accessing resources when using Maven
- Open Android Studio.
- In the project view, expand External Libraries.
- Scroll to the jwplayer-core module.
- Expand the jwplayer-core module and navigate to res/values.
- Double click values.xml.
- You now have access to the original style definitions used in the SDK.
Configuring the UI
Hiding UI Elements
There are two ways to hide UI Elements:
- Use the UiConfig to hide an entire class of UI elements (for example, hide NextUpView)
- Overwrite the style to hide individual UI elements (for example, hide Fullscreen icon)
Hide views with UiConfig
To hide the NextUpView, load the following UiConfig into the PlayerConfig:
UiConfig uiConfig = new UiConfig.Builder()
.displayAllControls()
.hide(UiGroup.NEXT_UP)
.build();
PlayerConfig config = new PlayerConfig.Builder()
.uiConfig(uiConfig)
.build();
Hide views with style resources
To hide the Fullscreen icon in the ControlbarView, add this style to your app's styles.xml. Setting a height
and width
of 0 ensures the UI element remains hidden even when the ViewModel instructs it to be visible.
When overwriting the style defined by the SDK with your own, you remove all of the style properties defined by the SDK. Therefore you may need to add some of the defaults back.
<style name="JWIcon.ControlbarFullscreen">
<item name="android:layout_height">0dp</item>
<item name="android:layout_width">0dp</item>
</style>
Changing Icons
To change the icon used in certain UI elements you can overwrite the drawable resource, ex. ic_jw_next.
Change icon with drawable resources
To change the icon for ic_jw_next, create an xml resource in the drawables folder of your app, name it ic_jw_next and place the desired icon resource within it.
<vector xmlns:android="http://schemas.android.com/apk/res/android"
android:width="24dp"
android:height="24dp"
android:viewportWidth="24.0"
android:viewportHeight="24.0">
<path
android:fillColor="#FF000000"
android:pathData="M6,18l8.5,-6L6,6v12zM16,6v12h2V6h-2z"/>
</vector>
Changing Colors
To change the colors used in certain UI elements you can:
- Overwrite the colors resource (for example, jw_icons_active)
- Overwrite the style to change the property (for example, android:textColor)
Change color with color resources
To change the icon for jw_icons_active, add a color in your app's colors.xml and name it jw_icons_active.
<color name="jw_icons_active">#A7EDA2</color>
Change color with style resources
To change the text color of the current position timestamp in the control bar, add the style to your app's styles.xml.
When overwriting the style defined by the SDK with your own, you remove all of the style properties defined by the SDK. Therefore you may need to add some of the defaults back.
<style name="JWText.ControlbarPosition">
<item name="android:layout_height">wrap_content</item>
<item name="android:layout_width">wrap_content</item>
<item name="android:textColor">#A7EDA2</item>
</style>
Changing Backgrounds
To change the background used in certain UI elements you can:
- Overwrite the drawable resource (for example, bg_jw_menu)
- Overwrite the style to change the property (for example, android:background)
Change background with drawable resources
To change the background for all menus, add a drawable in your app's drawable folder and name it bg_jw_menu.
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="#A7EDA2" />
<corners
android:bottomLeftRadius="0dp"
android:bottomRightRadius="0dp"
android:topLeftRadius="10dp"
android:topRightRadius="10dp" />
</shape>
Change background with style resources
To change the background of the Casting Menu specifically, add the style to your app's styles.xml.
When overwriting the style defined by the SDK with your own, you remove all of the style properties defined by the SDK. Therefore you may need to add some of the defaults back.
<style name="JWView.Menu.Cast">
<item name="android:background">#A7EDA2</item>
</style>
Changing Text
To change the text used in certain UI elements, you can overwrite the string resource (for example, jwplayer_next_up_countdown)
Change text with string resources
To change the Learn More text shown during a VAST ad, add a string in your app's strings.xml and name it jwplayer_learn_more.
<string name="jwplayer_learn_more">Click Me!!!</string>
Changing the size of UI Elements
To change the size of certain UI elements you can:
- Overwrite the dimen resource (for example, jw_large_icon)
- Overwrite the style to change the property (for example, android:layout_height)
Change size with dimen resources
To change the size of all of the main center controls, add a dimen in your app's dimens.xml and name it jw_large_icon.
<dimen name="jw_large_icon">80dp</dimen>
Change size with style resources
To change the size of the center combo box in CenterControlsView containing the Play/Pause/Buffer/Repeat Icon but not the other main controls, add the style to your app's styles.xml.
<style name="JWContainer.CenterControlsCombo">
<item name="android:layout_width">100dp</item>
<item name="android:layout_height">100dp</item>
</style>
UI Styling Reference
Controls
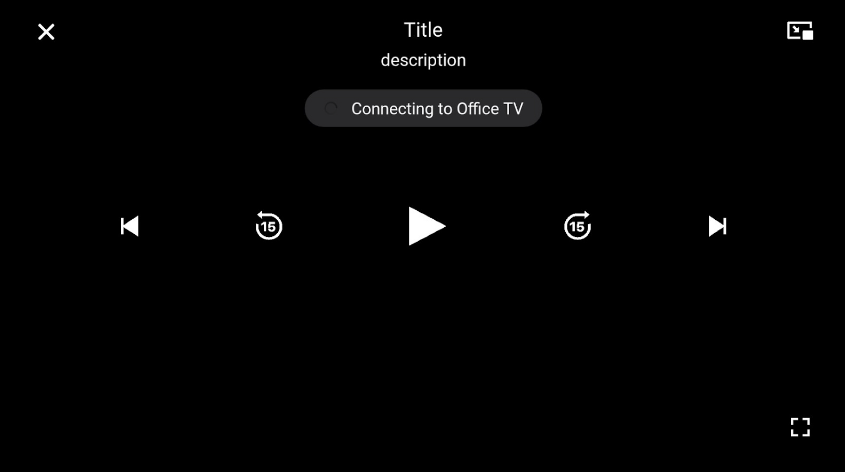
UiGroup.CENTER_CONTROLS
CenterControlsView
Icon | Resource Name | Colors | Dimens | Style | Description |
---|---|---|---|---|---|
![]() |
@drawable/bg_jw_center_controls |
@color/jw_controls_overlay |
N/A | JWView.CenterControls |
The CenterControls background |
![]() |
@drawable/ic_jw_play |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_large_icon |
JWIcon.CenterControlsPlay |
Play icon, shown when the player is Paused or Idle |
![]() |
@drawable/ic_jw_pause |
@color/jw_icons_active |
@color/jw_icons_tint @dimen/jw_large_icon |
JWIcon.CenterControlsPause |
Pause icon, shown when the player is Playing |
![]() |
@drawable/ic_jw_buffer |
@color/jw_buffer_start @color/jw_buffer_end |
@dimen/jw_large_icon |
JWIcon.CenterControlsBuffer |
Buffer spinner, shown when the player is Buffering |
![]() |
@drawable/ic_jw_replay |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_large_icon |
JWIcon.CenterControlsRepeat |
Replay icon, shown when the player has completed playback |
![]() |
@drawable/ic_jw_rewind_15 |
@color/jw_icons_active @color/jw_icons_inactive @color/jw_icons_tint |
@dimen/jw_large_icon |
JWIcon.CenterControlsRewind |
Rewind 15 seconds icon, shown when the player is playing seekable content |
![]() |
@drawable/ic_jw_fast_forward_15 |
@color/jw_icons_active @color/jw_icons_inactive @color/jw_icons_tint |
@dimen/jw_large_icon |
JWIcon.CenterControlsForward |
Fast Forward 15 seconds icon, shown when the player is playing seekable content |
![]() |
@drawable/ic_jw_next |
@color/jw_icons_active @color/jw_icons_inactive @color/jw_icons_tint |
@dimen/jw_large_icon |
JWIcon.CenterControlsNext |
Next playlist item icon, shown when the player has a playlist loaded |
![]() |
@drawable/ic_jw_cast_off |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.CenterControlsCast |
Cast icon (standby), shown when the player is configured with the cast module. |
![]() |
@drawable/ic_jw_cast_on |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.CenterControlsCast |
Cast icon (casting), shown when the player is actively casting media. |
![]() |
@drawable/ic_jw_pip |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.CenterControlsPip |
PiP icon, shown when the system supports PiP |
![]() |
@drawable/ic_jw_close |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.CenterControlsClose |
Close icon, shown when the player is in fullscreen mode. Clicking it exits fullscreen |
![]() |
@drawable/ic_jw_enter_fullscreen |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.CenterControlsFullscreen |
Enter fullscreen icon, shown when the player is not in fullscreen |
![]() |
@drawable/ic_jw_exit_fullscreen |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.CenterControlsFullscreen |
Exit fullscreen icon, shown when the player is in fullscreen |
![]() |
N/A | @color/jw_labels_primary |
N/A | JWText.CenterControlsTitle |
Displays the title of the current playlist item |
![]() |
N/A | @color/jw_labels_primary |
N/A | JWText.CenterControlsDescription |
Displays the description of the current playlist item |
Shown when the player is actively interacting with a cast device.

Connecting to {device} HUD, shown when the player is trying to connect to the cast device
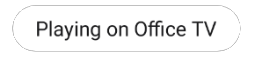
Playing on {device} HUD, shown when the player is actively casting to the device
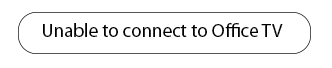
Unable to connect to {device} HUD, shown when the player could not connect the cast device
Icon | Resource Name | Colors | Dimens | Style | Description |
---|---|---|---|---|---|
![]() |
@drawable/bg_jw_cast_connecting |
@color/jw_fill_primary |
N/A | JWContainer.CenterControlsCastIndicator |
The background resource for the casting HUD when Connecting to the device |
![]() |
@string/jwplayer_cast_connecting_to |
@color/jw_labels_primary |
N/A | JWText.CenterControlsCastStatus |
The string resource used for the casting HUD when Connecting to the device |
![]() |
@drawable/bg_jw_cast_ready |
@color/jw_labels_primary |
N/A | JWContainer.CenterControlsCastIndicator |
The background resource for the casting HUD when actively playing on the cast device |
![]() |
@string/jwplayer_cast_playing_on |
@color/jw_surface_secondary |
N/A | JWText.CenterControlsCastStatus |
The string resource used for the casting HUD when actively playing on the cast device |
![]() |
@drawable/bg_jw_cast_ready |
@color/jw_labels_primary |
N/A | JWContainer.CenterControlsCastIndicator |
The background resource for the casting HUD when the player is unable to connect to the cast device |
@string/jwplayer_cast_unable_to_connect_to |
@color/jw_surface_secondary |
N/A | JWText.CenterControlsCastStatus |
The string resource used for the casting HUD when unable to connect to the cast device |
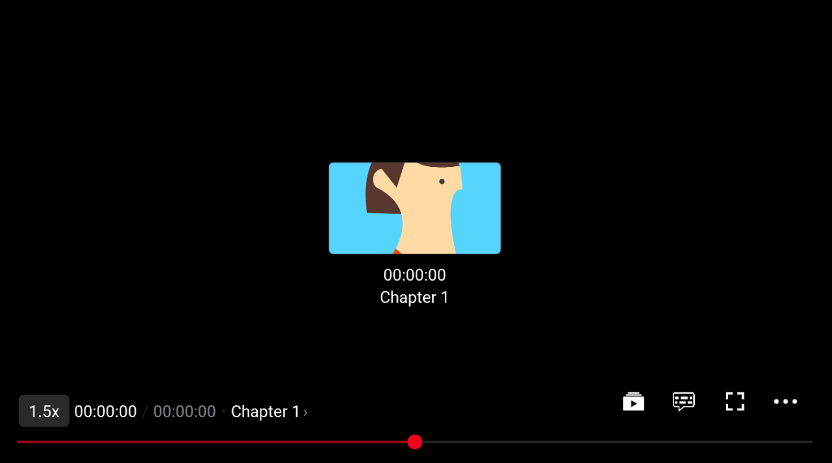
UiGroup.CONTROLBAR
ControlbarView
Icon | Resource Name | Colors | Dimens | Style | Description |
---|---|---|---|---|---|
@drawable/bg_jw_controlbar_contrast_gradient |
@color/jw_transparent @color/jw_controlbar_gradient_dark |
N/A | JWView.Controlbar |
The Controlbar background |
|
@drawable/bg_jw_controlbar_rates |
@color/jw_fill_primary |
@dimen/jw_icon @dimen/jw_text_medium |
JWText.ControlbarRate |
Playback speed indicator, shown when the player is playing content at a speed other that 1x |
|
![]() |
N/A | @color/jw_labels_primary |
@dimen/jw_text_medium |
JWText.ControlbarPosition |
Current playback position timestamp |
@string/jwplayer_control_bar_timer_delimiter |
@color/jw_surface_secondary |
@dimen/jw_text_medium |
JWText.ControlbarPositionDivider |
Separator between position and duration |
|
N/A | @color/jw_labels_secondary |
@dimen/jw_text_medium |
JWText.ControlbarDuration |
Current video's duration |
|
@string/jwplayer_dot_separator |
@color/jw_surface_secondary |
@dimen/jw_text_medium |
JWText.ControlbarChaptersDivider |
Separator between duration and chapter |
|
N/A | @color/jw_labels_primary |
@dimen/jw_text_medium |
JWText.ControlbarChaptersTitle |
Name of the current chapter. Tapping on this will open the Chapters menu |
|
@string/jwplayer_arrow_indicator |
@color/jw_labels_secondary |
@dimen/jw_text_medium |
JWText.ControlbarChaptersIndicator |
Indicator to show that more chapters exist |
|
@drawable/ic_jw_captions_off |
@color/jw_icons_active @color/jw_icons_inactive @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.ControlbarCaptions |
Closed captions toggle. Tapping this will toggle captions if only one track exists or open the captions menu if more exist. This icon indicates that the captions are off |
|
@drawable/ic_jw_captions_on |
@color/jw_icons_active @color/jw_icons_inactive @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.ControlbarCaptions |
This icon indicates that the captions are on |
|
@drawable/ic_jw_more_videos |
@color/jw_icons_active @color/jw_icons_inactive @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.ControlbarPlaylist |
Playlist icon, tapping this opens the playlist view |
|
@drawable/ic_jw_enter_fullscreen |
@color/jw_icons_active @color/jw_icons_inactive @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.ControlbarFullscreen |
Enter fullscreen icon, tapping this puts the player in fullscreen |
|
@drawable/ic_jw_exit_fullscreen |
@color/jw_icons_active @color/jw_icons_inactive @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.ControlbarFullscreen |
Exit fullscreen icon, tapping this takes the player out of fullscreen |
|
@drawable/ic_jw_settings |
@color/jw_icons_active @color/jw_icons_inactive @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.ControlbarMenu |
Settings icon, tapping this opens the settings menu |
|
@string/jwplayer_live_broadcast |
@color/jw_labels_primary |
@dimen/jw_text_xsmall |
JWRadio.ControlbarLive |
Live Indicator |
|
@drawable/jw_controlbar_live_button |
@color/jw_live_indicator_checked @color/jw_live_indicator_unchecked |
N/A | JWRadio.ControlbarLive |
Live Indicator |
|
N/A | N/A | N/A | JWControlbarSeek |
Timeslider |
|
N/A | @color/jw_seekbar_progress |
@dimen/jw_seekbar_thickness |
JWControlbarSeek |
The current progress of the seekbar |
|
N/A | @color/jw_seekbar_ads_marker |
@dimen/jw_seekbar_ad_width @dimen/jw_seekbar_thickness |
JWControlbarSeek |
Marker signifying an ad was scheduled in the timeline |
|
N/A | @color/jw_seekbar_chapter_marker |
@dimen/jw_seekbar_chapter_width @dimen/jw_seekbar_thickness |
JWControlbarSeek |
Marker signifying a chapter begins |
|
N/A | @color/jw_seekbar_chapter_highlight |
@dimen/jw_seekbar_thickness |
JWControlbarSeek |
Highlights the timeline between the start of the current chapter and the start of the next |
|
@drawable/jw_controlbar_seek_thumb |
@color/jw_seekbar_thumb |
@dimen/jw_seekbar_thumb |
JWControlbarSeek |
The seekbar thumb |
|
N/A | @color/jw_seekbar_secondary_progress |
@dimen/jw_seekbar_thickness |
JWControlbarSeek |
The seekbar secondary progress, this indicates the amount of buffered video |
|
N/A | @color/jw_seekbar_background |
@dimen/jw_seekbar_thickness |
JWControlbarSeek |
The seekbar background, this indicates the size of the seekbar |
|
N/A | @color/jw_fill_primary |
N/A | JWImage.ControlbarThumbnailPreview JWRoundedImageView.ThumbnailPreview |
Thumbnail preview, updates the bitmap based on the position you are scrubbing. |
|
![]() |
N/A | @color/jw_labels_primary |
@dimen/jw_text_medium |
JWText.ControlbarThumbnailTimestamp |
The position you are scrubbing to |
N/A | @color/jw_labels_primary |
@dimen/jw_text_medium |
JWText.ControlbarThumbnailChapter |
The chapter you are scrubbing to |
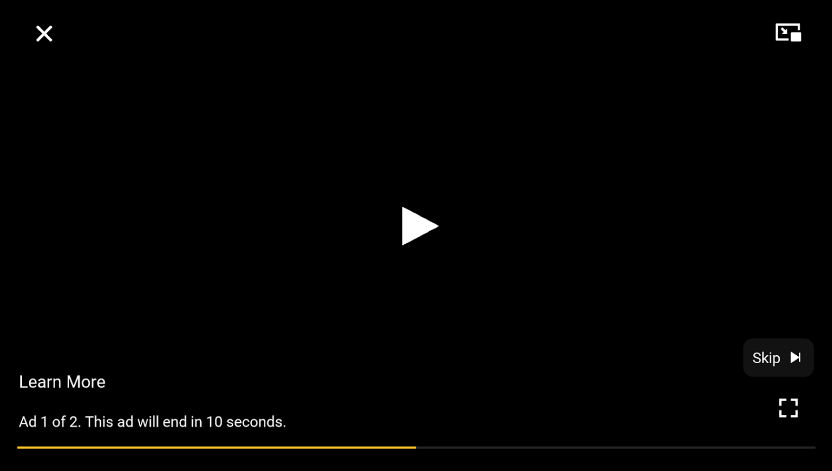
UiGroup.ADS_CONTROL
VastAdsView
Icon | Resource Name | Colors | Dimens | Style | Description |
---|---|---|---|---|---|
N/A | @color/jw_controls_overlay |
N/A | N/A | The vast controls background |
|
@drawable/ic_jw_play |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_large_icon |
JWIcon.VastPlay |
Play icon, tapping it resumes the ad |
|
@drawable/ic_jw_pause |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_large_icon |
JWIcon.VastPlay |
Pause icon, tapping it pauses the ad |
|
@string/jwplayer_learn_more |
@color/jw_labels_primary |
@dimen/jw_text_large |
JWText.VastLearnMore |
Learn More button, tapping it redirects the user to the ad's clickthrough URL |
|
@string/jwplayer_advertising_ad_x_of_y @string/jwplayer_advertising_remaining_time |
@color/jw_labels_primary |
@dimen/jw_text_small |
JWText.VastAdMessage |
Ad message, configurable via the string resources. |
|
@drawable/jw_vast_seek_progress_drawable |
@color/jw_vast_progress |
@dimen/jw_seekbar_thickness |
VastProgress |
Ad progress |
|
@drawable/jw_vast_seek_progress_drawable |
@color/jw_seekbar_background |
@dimen/jw_seekbar_thickness |
VastProgress |
Progressbar background |
|
@drawable/bg_jw_vast_skip @drawable/ic_jw_skip |
@color/jw_surface_secondary_non_opaque @color/jw_icons_active @color/jw_labels_primary |
@dimen/jw_text_small |
JWText.VastSkip |
Skip button |
|
@drawable/ic_jw_close |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.VastClose |
Exit fullscreen icon, shown when the player is in fullscreen, tapping it exits fullscreen |
|
@drawable/ic_jw_pip |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.VastPip |
PiP icon, tapping it puts the player/activity in PiP mode |
|
@drawable/ic_jw_enter_fullscreen |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.VastFullscreen |
Enter fullscreen icon, shown when the player is not in fullscreen, tapping it enters fullscreen |
|
@drawable/ic_jw_exit_fullscreen |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.VastFullscreen |
Exit fullscreen icon, shown when the player is in fullscreen, tapping it exits fullscreen |
Menus
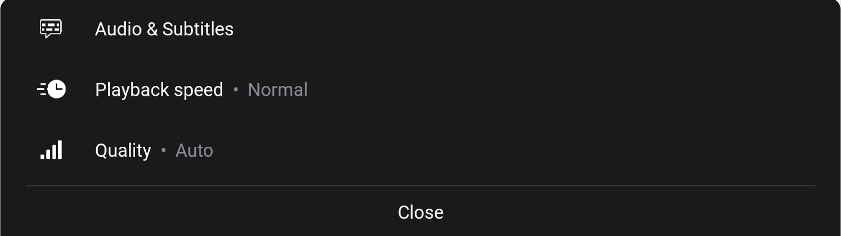
UiGroup.SETTINGS_MENU
MenuView
Icon | Resource Name | Colors | Dimens | Style | Description |
---|---|---|---|---|---|
@drawable/bg_jw_menu |
@color/jw_surface_secondary |
N/A | JWView.Menu.Settings |
The menu background |
|
@drawable/ic_jw_captions_off |
@color/jw_icons_active @color/jw_icons_tint |
N/A | JWIcon.MenuOption |
Icon for alternate audio and captions. Tapping this takes you to the Audio & Subtitles submenu |
|
@string/jwplayer_audio_and_subtitles |
N/A | @dimen/jw_text_xlarge |
JWText.MenuOptionLabel |
Label for the Audio & Subtitles submenu |
|
@drawable/ic_jw_play |
@color/jw_icons_active @color/jw_icons_tint |
N/A | JWIcon.MenuOption |
Icon for playback speed. Tapping this takes you to the Playback speed submenu |
|
@string/jwplayer_playback_rates |
@color/jw_labels_primary |
@dimen/jw_text_xlarge |
JWText.MenuOptionLabel |
Label for the Playback Speed submenu |
|
N/A | @color/jw_labels_secondary |
@dimen/jw_text_xlarge |
JWText.MenuOptionValue |
Indicator showing the current Playback Speed |
|
@drawable/ic_jw_quality_100 |
@color/jw_icons_active @color/jw_icons_tint |
N/A | JWIcon.MenuOption |
Icon for video quality. Tapping this takes you to the qualities submenu |
|
@string/jwplayer_quality |
@color/jw_labels_primary |
@dimen/jw_text_xlarge |
JWText.MenuOptionLabel |
Label for the Quality submenu |
|
N/A | @color/jw_labels_secondary |
@dimen/jw_text_xlarge |
JWText.MenuOptionValue |
Indicator showing the current Quality |
|
N/A | @color/jw_separator_non_opaque |
N/A | JWHorizontalDivider.Menu |
Divider meant to divide the list from the close button |
|
@string/jwplayer_close |
@color/jw_labels_primary |
@dimen/jw_text_xlarge @dimen/jw_icon |
JWText.MenuClose |
Close button |
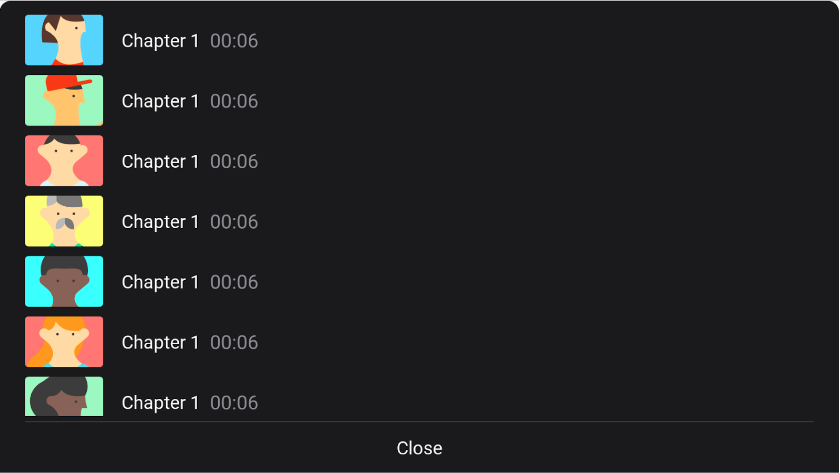
UiGroup.CHAPTERS
ChaptersView
Icon | Resource Name | Colors | Dimens | Style | Description |
---|---|---|---|---|---|
@drawable/bg_jw_menu |
@color/jw_surface_secondary |
N/A | JWView.Menu.Chapters |
The menu background |
|
N/A | N/A | @dimen/jw_icon |
JWImage.ChaptersItemPoster JWRoundedImageView.Chapters |
The thumbnail of the chapter. Derived from the chapters VTT file |
|
N/A | @color/jw_labels_primary |
@dimen/jw_text_xlarge |
JWText.ChaptersItemTitle |
Name of the chapter |
|
N/A | @color/jw_labels_secondary |
@dimen/jw_text_xxlarge |
JWText.ChaptersItemPosition |
Time the chapter begins |
|
N/A | @color/jw_separator_non_opaque |
N/A | JWHorizontalDivider.Chapters |
Divider meant to divide the list from the close button |
|
@string/jwplayer_close |
@color/jw_labels_primary |
@dimen/jw_text_xlarge @dimen/jw_icon |
JWText.ChaptersClose |
Close button |
Overlays
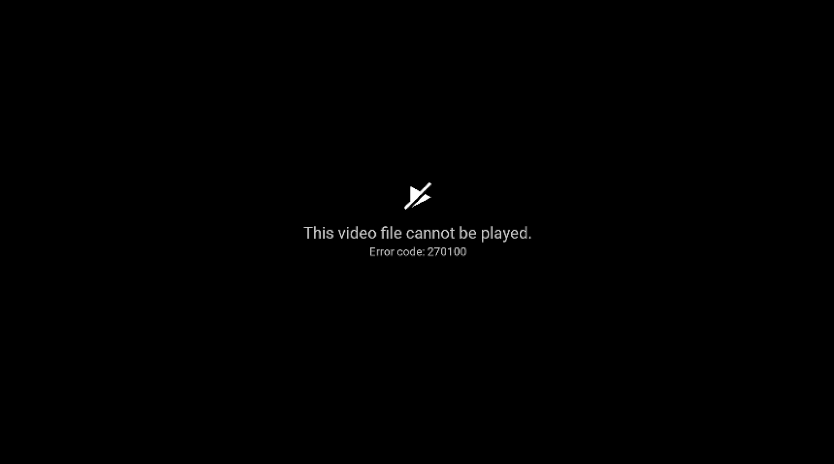
UiGroup.ERROR
ErrorView
Icon | Resource Name | Colors | Dimens | Style | Description |
---|---|---|---|---|---|
@drawable/bg_jw_error |
@color/jw_surface_primary |
N/A | JWContainer.Error |
The error background |
|
@drawable/ic_jw_play |
@color/jw_icons_active |
@dimen/jw_large_icon |
JWImage.ErrorIcon |
Error indicator |
|
N/A | @color/jw_labels_primary |
@dimen/jw_text_medium |
JWText.ErrorMessage |
Error message |
|
N/A | @color/jw_labels_secondary |
@dimen/jw_text_xxsmall |
JWText.ErrorCode |
Error code |
Playlists
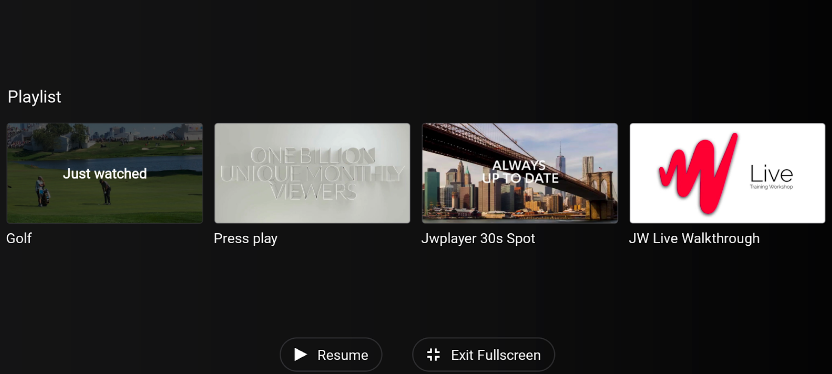
UiGroup.PLAYLIST
PlaylistView
Icon | Resource Name | Colors | Dimens | Style | Description |
---|---|---|---|---|---|
@drawable/bg_jw_playlist |
@color/jw_surface_secondary |
N/A | JWContainer.Playlist |
The playlist background |
|
@string/jwplayer_playlist |
@color/jw_labels_primary |
@dimen/jw_text_xxlarge |
JWText.PlaylistHeader |
Playlist heading, indicates that the items currently visible are part of a playlist |
|
@drawable/bg_jw_playlist_button_border |
@color/jw_fill_primary |
@dimen/jw_thin_border |
JWText.PlaylistResume |
Resume button border |
|
@drawable/ic_jw_play |
@color/jw_icons_active |
N/A | JWText.PlaylistResume |
Resume button Play icon |
|
@string/jwplayer_resume |
@color/jw_labels_primary |
@dimen/jw_text_medium |
JWText.PlaylistResume |
Resume button text resource |
|
@drawable/bg_jw_playlist_button_border |
@color/jw_fill_primary |
@dimen/jw_thin_border |
JWText.PlaylistExitFullscreen |
Exit fullscreen button border |
|
@drawable/ic_jw_play |
@color/jw_icons_active |
N/A | JWText.PlaylistExitFullscreen |
Exit fullscreen icon |
|
@string/jwplayer_exit_fullscreen |
@color/jw_labels_primary |
@dimen/jw_text_medium |
JWText.PlaylistExitFullscreen |
Exit Fullscreen text resource |
|
N/A | @color/jw_fill_primary |
N/A | JWImage.PlaylistItemPoster JWRoundedImageView.Playlist |
The playlist item's poster image as defined by the metadata provided to the player |
|
@string/jwplayer_just_watched @drawable/bg_jw_playlist_item_poster_overlay |
@color/jw_labels_primary |
@dimen/jw_text_medium |
JWText.PlaylistItemWatched |
Just Watched, indicates the current playlist item |
|
@drawable/bg_jw_playlist_item_duration |
@color/jw_labels_primary |
@dimen/jw_text_xsmall |
JWText.PlaylistItemDuration |
Duration of the playlist item as defined by the metadata provided to the player |
|
N/A | @color/jw_labels_primary |
@dimen/jw_text_medium |
JWText.PlaylistItemTitle |
Playlist item's title as defined by the metadata provided to the player |
|
@string/jwplayer_recommendations |
@color/jw_labels_primary |
@dimen/jw_text_xxlarge |
JWText.PlaylistHeader |
Recommendations heading, indicates that the items currently visible are recommended media |
|
N/A | @color/jw_autoplay_progress @color/jw_autoplay_background |
N/A | JWIcon.PlaylistNextUpCircularProgress |
Progress bar indicating the countdown to the next playlist item |
|
@drawable/ic_jw_play |
@color/jw_icons_active @color/jw_icons_tint |
N/A | JWIcon.PlaylistNextUpPlay |
Play icon |
|
@string/jwplayer_next_up_countdown |
@color/jw_labels_primary |
@dimen/jw_text_medium |
JWText.PlaylistNextUpCountdown |
Countdown indicating that the next video is about to begin |
|
N/A | @color/jw_labels_primary |
@dimen/jw_text_medium |
JWText.PlaylistNextUpTitle |
The title of the next playlist item as defined by the metadata provided to the player |
|
@drawable/bg_jw_playlist_poster_gradient |
@color/jw_recs_gradient_light @color/jw_recs_gradient_mid @color/jw_recs_gradient_dark |
N/A | JWContainer.PlaylistBackgroundPosterGradient |
Gradient overlay on top of the next up playlist item poster image |
|
N/A | N/A | N/A | JWImage.PlaylistBackgroundPoster |
Poster image for the next up playlist item as defined by the metadata provided to the player |
|
@drawable/ic_jw_more_videos @drawable/bg_jw_more_videos |
@color/jw_icons_active @color/jw_icons_tint @color/jw_fill_primary |
N/A | JWIcon.PlaylistMoreVideos |
More videos button, shows the recommendations feed when a playlist is loaded and recommendations are defined. |
|
@string/jwplayer_more_videos |
@color/jw_labels_primary |
N/A | JWText.PlaylistMoreVideos |
More videos label |

UiGroup.NEXT_UP
NextUpView
Icon | Resource Name | Colors | Dimens | Style | Description |
---|---|---|---|---|---|
@drawable/bg_jw_next_up |
@color/jw_surface_secondary |
@dimen/jw_nextup_view_height |
JWView.NextUp |
The NextUp background |
|
N/A | @color/jw_fill_primary |
N/A | JWImage.NextUpPoster |
Poster image for the next up playlist item as defined by the metadata provided to the player. |
|
@string/jwplayer_next_up_countdown |
@color/jw_labels_primary |
N/A | JWText.NextUpCountdown |
Countdown indicating that the next video is about to begin |
|
N/A | @color/jw_labels_primary |
N/A | JWText.NextUpTitle |
The title of the next playlist item as defined by the metadata provided to the player |
|
@drawable/ic_jw_close @drawable/bg_jw_nextup_close |
@color/jw_icons_active @color/jw_icons_tint @color/jw_fill_quaternary |
N/A | JWIcon.NextUpClose |
Dismiss button, dismisses the next up prompt |
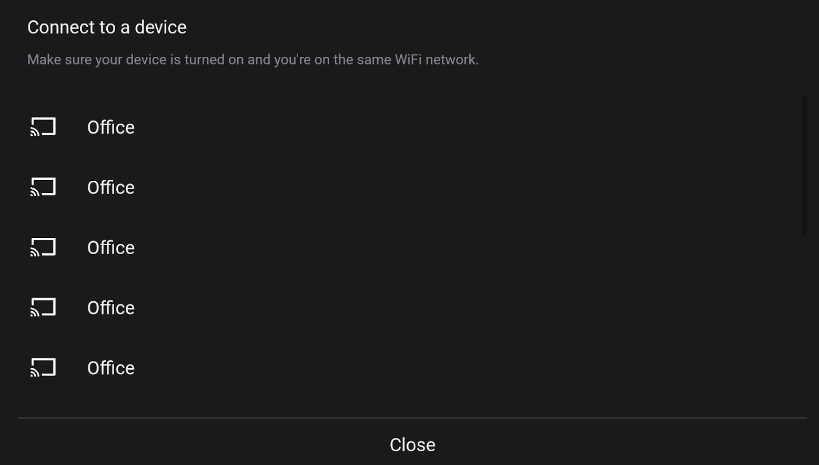
UiGroup.CASTING_MENU
CastingMenuView
Icon | Resource Name | Colors | Dimens | Style | Description |
---|---|---|---|---|---|
@drawable/bg_jw_menu |
@color/jw_surface_secondary |
N/A | JWView.Menu.Cast |
The menu background |
|
@string/jwplayer_cast_connect_to_a_device |
@color/jw_labels_primary |
@dimen/jw_text_xlarge |
JWText.CastTitle |
Title and call to action for the casting menu |
|
@string/jwplayer_cast_use_same_wifi |
@color/jw_labels_secondary |
@dimen/jw_text_xsmall |
JWText.CastSubtitle |
Hint that your mobile device needs to be connected to the same network as the cast device |
|
@drawable/ic_jw_cast_off |
@color/jw_icons_active @color/jw_icons_tint |
@dimen/jw_icon |
JWIcon.CastItem |
Icon to indicate a cast device |
|
N/A | @color/jw_labels_primary |
@dimen/jw_text_xlarge |
JWText.CastItem |
Name of the cast device |
|
N/A | @color/jw_separator_non_opaque |
N/A | JWHorizontalDivider.Cast |
Divider |
|
@string/jwplayer_close |
@color/jw_labels_primary |
@dimen/jw_text_xlarge @dimen/jw_icon |
JWText.CastClose |
Close button |
CastDialog
Icon | Resource Name | Colors | Dimens | Style | Description |
---|---|---|---|---|---|
@drawable/bg_jw_cast_disconnect_dialog |
@color/jw_surface_quaternary |
N/A | JWView.CastDialog |
The Cast Dialog background |
|
@string/jwplayer_cast_playing_on |
@color/jw_labels_primary |
@dimen/jw_text_large |
JWText.CastDialogDevice |
Indicates which device you are currently casting to |
|
@string/jwplayer_cancel |
@color/jw_labels_primary |
@dimen/jw_text_large |
JWText.CastDialogCancel |
Closes the Cast Disconnect Dialog |
|
@string/jwplayer_cast_disconnect |
@color/jw_labels_primary |
@dimen/jw_text_large |
JWText.CastDialogDisconnect |
Disconnects from the casting device and resumes playback on the mobile device |
Updated 6 months ago